SALV: ALV List Quickstart
Snippets to get your ALV Grid up and running in no time 🚀
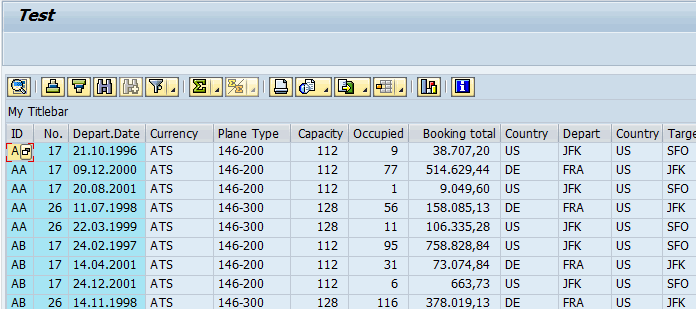
Context
The object model for the SAP List Viewer (ALV) is an object-oriented encapsulation of SAP’s much older ALV tool.
As of today class CL_SALV_TABLE
remains widely used for displaying simple, two-dimensional ALV grids.
Below is a collection of short snippets covering the most basic requirements concerning ALVs made via this class.
Note: For simplicity’s sake error handling of SALV errors (subclasses of exception class CX_SALV_ERROR
) have been left out. Make sure to implement appropriate exception handling for productive code.
Basics
"Instantiation
cl_salv_table=>factory(
IMPORTING
r_salv_table = DATA(lo_alv)
CHANGING
t_table = mt_data ).
"Do stuff
"...
"Display the ALV Grid
lo_alv->display( ).
Column settings
Change header text
DATA(lo_column) = lo_alv->get_columns( )->get_column( 'COLUMNNAME' ).
lo_column->set_long_text( 'LONG_HEADER' ).
lo_column->set_medium_text( 'MEDIUM_HEADER' ).
lo_column->set_short_text( 'SHORT_HEADER' ).
Optimize column width
lo_alv->get_columns( )->set_optimize( abap_true ).
Sort columns
"Sort a column up
lo_alv->get_sorts( )->add_sort( 'COLUMNNAME' ).
Hotspots
About
Hotspots are special cells of an ALV list that can be compared to hyperlinks or buttons on web pages/apps. If a user clicks once onto a hotspot field an event is triggered. For fields that are not defined as hotspots, the user must double-click the field or use a function key to trigger an event.
Register hotspot column
DATA(lo_column_tab) = CAST cl_salv_column_table(
lo_alv->get_columns( )->get_column( 'COLUMNNAME' ) ).
lo_column_tab->set_cell_type( if_salv_c_cell_type=>hotspot ).
Register hotspot click handler
DATA(lo_events) = lo_alv->get_event( ).
SET HANDLER lcl_application->on_link_click FOR lo_events.
Handler implementation
CLASS lcl_application DEFINITION.
PRIVATE SECTION.
METHODS on_link_click
FOR EVENT link_click OF cl_salv_events_table
IMPORTING
row
column.
"...
ENDCLASS.
CLASS lcl_application IMPLEMENTATION.
METHOD on_link_click.
"Handle hotspot click
ENDMETHOD.
ENDCLASS.
Aggregations
Class CL_SALV_AGGREGATIONS
can be used to display basic statistical facts for a given column.
Options include displaying the total, minimum or maximum, and average value.
"Show total for column 'COLUMNNAME'
DATA(lo_aggregations) = lo_alv->get_aggregations( ).
lo_aggregations->add_aggregation(
columnname = 'COLUMNNAME'
aggregation = if_salv_c_aggregation=>total ).
List selections
Set selection mode
DATA(lo_selections) = lo_alv->get_selections( ).
"allow single line selection
lo_selections->set_selection_mode( if_salv_c_selection_mode=>single ).
Get selected lines
DATA(lo_selections) = lo_alv->get_selections( ).
DATA(lt_selected_row_indices) = lo_selections->get_selected_rows( ).
IF lines( lt_selected_row_indices ) NE 1.
"Select one row for this function
MESSAGE s022(upp_pp_k) DISPLAY LIKE 'W'.
RETURN.
ENDIF.
READ TABLE lt_selected_row_indices INDEX 1 INTO DATA(lv_selected_row_index).
READ TABLE mt_data
INDEX lv_selected_row_index
ASSIGNING FIELD-SYMBOL(<s_selected_row>).
IF sy-subrc NE 0.
"Select one row for this function
MESSAGE s022(upp_pp_k) DISPLAY LIKE 'W'.
RETURN.
ENDIF.
"Handle user action
"...
Variants
Initialize: set layout key
DATA(lo_layout) = lo_alv->get_layout( ).
"set layout key for identifying the particular ALV list
lo_layout->set_key( VALUE #( report = sy-repid ) ).
Allow variant saving
"remove restriction on saving layouts
lo_alv->get_layout( )->set_save_restriction( if_salv_c_layout=>restrict_none ).
Set initial variant
"set initial layout
lo_alv->get_layout( )->set_initial_layout( 'VARIANT_NAME' ).
Allow default variants
"allow setting layouts as default layouts
lo_alv->get_layout( )->set_default( abap_true ).
Example: setting up ALV variants
METHODS set_layout
IMPORTING io_alv TYPE REF TO cl_salv_table
RAISING cx_salv_error.
METHOD set_layout.
DATA(lo_layout) = io_alv->get_layout( ).
"set layout key for identifying the particular ALV list
lo_layout->set_key( VALUE #( report = sy-repid ) ).
"remove restriction on saving layouts
lo_layout->set_save_restriction( if_salv_c_layout=>restrict_none ).
"allow setting layouts as default layouts
lo_layout->set_default( abap_true ).
ENDMETHOD.
Handling ALV toolbar functions
Default functions
"Enable default ALV toolbar functions
lo_alv->get_functions( )->set_default( abap_true ).
Custom functions
Creating a custom GUI status
Instead of manually creating a GUI status we can copy the status SALV_STANDARD
of demo program
SALV_DEMO_TABLE_FUNCTIONS
to quickly get things up and running.
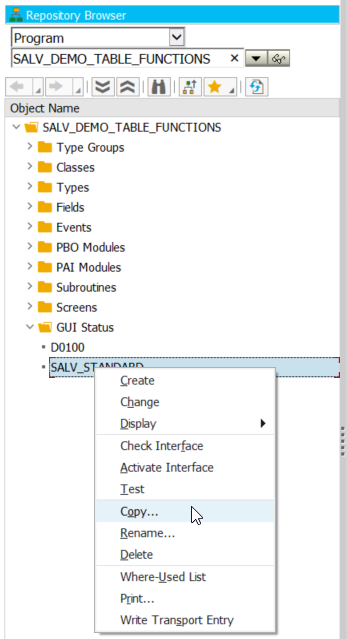
SALV_DEMO_TABLE_FUNCTIONS
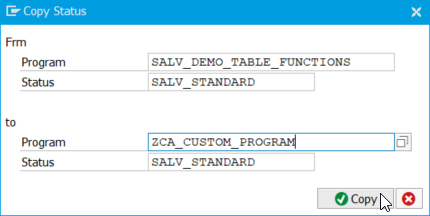
SALV_DEMO_TABLE_FUNCTIONS
to a custom programThis status includes all standard ALV functions as well as a demo custom function.

SALV_STANDARD
in demo program SALV_DEMO_TABLE_FUNCTIONS
The demo function’s attributes can be adjusted in the Menu Painter (via transaction SE80 or transaction SE41).
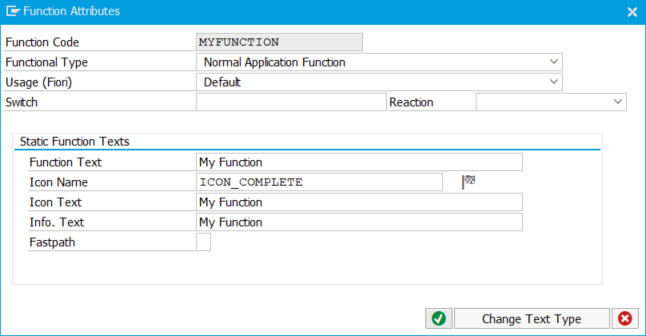
SALV_STANDARD
Register an ALV GUI status
CONSTANTS gui_status_name TYPE sypfkey VALUE 'SALV_STANDARD'.
"Register a custom GUI status for an ALV
lo_alv->set_screen_status(
pfstatus = gui_status_name
report = sy-repid
set_functions = lo_alv->c_functions_all ).
Handler method for custom functions
"Handler method for custom functions
METHODS on_user_command
FOR EVENT added_function OF cl_salv_events
IMPORTING !e_salv_function .
METHOD on_user_command.
CASE e_salv_function.
WHEN 'MYFUNCTION'.
"Handle custom function
"...
ENDCASE.
ENDMETHOD.
"Register handler for custom function
SET HANDLER on_user_command FOR lo_alv->get_event( ).
Coloring list data
Colors can help draw attention to outliers in the output data. For example, colors can bring negative balances or successfully handled records to the end user’s attention. We can colorize three different levels in the list output: cells, rows, and columns.
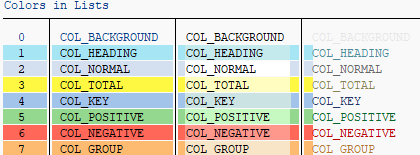
DEMO_LIST_FORMAT_COLOR_1
Colored cell/row
Add color column to ALV data structure, type lvc_t_scol
TYPES:
BEGIN OF ty_s_data,
carrid TYPE s_carr_id,
connid TYPE s_conn_id,
fldate TYPE s_date,
seatsocc TYPE s_seatsocc,
t_color TYPE lvc_t_scol, "Color column
END OF ty_s_data.
DATA mt_data TYPE TABLE OF ty_s_data.
Color a particular cell
"Color a particular cell based on a condition
LOOP AT mt_data ASSIGNING FIELD-SYMBOL(<s_data>) WHERE seatsocc > 300.
APPEND VALUE #(
fname = 'SEATSOCC'
color-col = col_negative
color-int = 0 "'Intensified' off
color-inv = 0 "'Inverse' off
) TO <s_data>-t_color.
ENDLOOP.
Color an entire row
"Color an entire row based on a condition
LOOP AT mt_data ASSIGNING <s_data> WHERE seatsocc < 100.
"Don't specify a field name in order to apply a color to a whole row
APPEND VALUE #( color-col = col_positive ) TO <s_data>-t_color.
ENDLOOP.
Register the color column
"Register the color column before displaying the ALV
lo_alv->get_columns( )->set_color_column( 'T_COLOR' ).
Colored column
DATA(lo_column_tab) = CAST cl_salv_column_table(
lo_alv->get_columns( )->get_column( 'COLUMNNAME' ) ).
"It's not possible to change the column color of key fields,
"so unregister the column as key field when needed
lo_column_tab->set_key( abap_false ).
lo_column_tab->set_color( VALUE #( col = col_positive ) ).